- From: Rick Waldron via GitHub <sysbot+gh@w3.org>
- Date: Fri, 05 Jun 2015 18:40:39 +0000
- To: public-device-apis@w3.org
@tobie > Is such an identifier the right solution? Yes, I think this is a reasonable approach. > Should we favor consistency or context? Subjectively, I like the consistent approach: ```js new Sensor.Proximity({ identifier: "back-right" }); new Sensor.Temperature({ identifier: "internal" }); ``` Because it's very clear what the intention of this data point is: "I want the back-right Proximity sensor and the internal Temperature sensor". It's easy to reason about. _However_, I have concerns about how to match "the one I need in this program" with whatever the `identifier` name is and whether or not this platform even has that one. > What happens when no identifier is passed to the constructor? Must there be a default sensor for each type so that you can do: new Sensor.Geoloc() and not new Sensor.Geoloc({ identifier: "default" })? Yes, if nothing is provided you get the default of that sensor, with the default settings for that component on that platform. This is probably just fine for most sensor types on most platforms and optimizes the written code for the most common case. Also, if user code does provide optional data properties to the constructor, but not an `identifier` property, then they will get the default with those options applied: ```js new Sensor.Temperature({ frequency: 5 }); // the default, but with a specified 5Hz frequency ``` > Where are those lists of sensors defined? In the concrete specs themselves? Through an API the consumer can query (e.g. Sensor.Proximity.getAll())? (Grouping this with response to @davidmarkclements) > `var pSensors = Sensor.Proximity.list();` Subjectively, `list` > `getAll`. ```js // If I apply node-serialport's "list()" to this spec, it would look like this: Sensor.Proximity.list(function(error, theList) { // theList is an array of objects containing meta data about each // Proximity sensor available on the device. }); // If I wrote it as a promise thing, it would be similar, but obviously a promise: Sensor.Proximity.list().then(function(theList) { // theList is an array of objects containing meta data about each // Proximity sensor available on the device }); ``` (I honestly don't care which, but I'm sure the latter will be the general favorite) To be certain, the operation has to be async, because even if the platform knows exactly what it has for hardware before the browser even opens this page, it might not know if the page is instructing the user to plug something in. We agreed that will be future-feature-work, but in the spirit of preventing a design-into-corner problem, it makes sense for it to start life as an async API. In the meantime, if a program needs to be specific about which sensor it wants to know about, then it's ok to require this "1-turn fee" to get that list. > //deliberately not using new Why? Not doing so will not work correctly. These are classes that instantiate new instances of a specific sensor object. `String(0)` returns a primitive string, not a `String` object, and `Map()` throws an exception because there is no such thing as a primitive `Map`—there is no exception here. ------------------ We'll need to specify a simple naming system for these meta details. To get us started: 6 direction surfaces with 9 position sections per surface, works well with any mobile device, watch, goggles, laptop keyboard surface, laptop screen surface, a traditional keyboard, a traditional monitor, a touch keyboard surface, a touch monitor, and likely many more. I will gladly provide diagrams if necessary. - Components that may have greater than one instance per device, eg. Ambient Light, Infrared (range and motion), Proximity, Temperature - position: One of ["top-left", "top", "top-right", "middle-left", "middle", "middle-right", "bottom-left", "bottom", "bottom-right"] - direction: One of ["front", "rear", "left", "right", "top", "bottom"] - internal: true | false - Components that will likely only have one per device, eg. Accelerometer, Altitude, Ambient Light (intentionally in both lists), Barometric pressure, Compass, Fingerprint reader, Geolocation (GPS + GSM), Gyroscope, Temperature (intentionally in both lists) - internal: true | false Terminology: #### direction - **front**: surface facing the user <img src="http://im.rediff.com/gadget/2015/mar/iwatch4.jpg" width="200"> <img src="http://g-ecx.images-amazon.com/images/G/01/electronics/apple/apple-12q2-macbook-pro-13-front-lg.jpg" width="200"> <img src="http://store.storeimages.cdn-apple.com/4589/as-images.apple.com/is/image/AppleInc/aos/published/images/i/ph/iphone6/plus/iphone6-plus-box-silver-2014?wid=478&hei=595&fmt=jpeg&qlt=95&op_sharpen=0&resMode=bicub&op_usm=0.5,0.5,0,0&iccEmbed=0&layer=comp&.v=1411520747267" width="100"> - **rear**: surface facing away from the user <img src="http://images.gizmag.com/inline/applewatch-9.jpg" width="200"> <img src="http://cdn.redmondpie.com/wp-content/uploads/2010/08/MacBookPro.jpg" width="200"> <img src="http://asset2.cbsistatic.com/cnwk.1d/sc/34117595-2-440-BK.jpg" width="200"> - **left**: the left "edge" of the device <img src="http://www.yogadz.com/wp-content/uploads/2013/09/iPhone-5c-side-by-side.jpg" width="200"> <img src="http://images.appleinsider.com/mb15alumunbox-081016-6.jpg" width="200"> - **right**: the right "edge" of the device <img src="http://d.fastcompany.net/multisite_files/fastcompany/imagecache/slideshow_large/slideshow/2014/09/3035366-slide-i-5-apple-watch.jpg" width="200"> <img src="http://1.bp.blogspot.com/-bmNxiBgbdD4/VCe_Im4-lLI/AAAAAAAAHyE/QhexWdOglws/s1600/Apple-iPhone-6-Plus-3.JPG" width="200"> - **top**: the top "edge" of the device - **bottom**: the bottom "edge" of the device <img src="http://gadgetstress.com/wp-content/uploads/2011/12/006-view-from-side.jpg" width="200"> Examples: 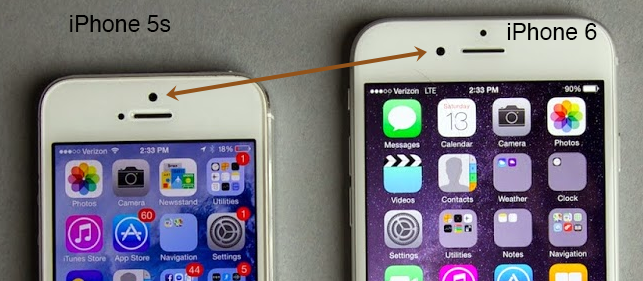 ``` // Proximity { direction: "front", position: "top", internal: false } ```  ``` // Proximity { direction: "front", position: "top-right", internal: false } ```  ``` // Accelerometer { internal: true } ```  ``` // Gyroscope { internal: true } ``` ------ 6 direction surfaces with 9 position sections per surface, works well with any mobile device, watch, goggles, laptop keyboard surface, laptop screen surface, a traditional keyboard, a traditional monitor, a touch keyboard surface, a touch monitor, and likely many more. -- GitHub Notif of comment by rwaldron See https://github.com/w3c/sensors/issues/26#issuecomment-109392164
Received on Friday, 5 June 2015 18:40:41 UTC