- From: guest271314 via GitHub <sysbot+gh@w3.org>
- Date: Sun, 02 Jun 2019 04:41:07 +0000
- To: public-webrtc-logs@w3.org
A rudimentary POC https://plnkr.co/edit/UmrSwN?p=preview to select only part of a screen for a screen shot (the movement and resizing of the selection element can be fine-tuned to have similar functionality as, for example, https://codepen.io/zz85/pen/gbOoVP) ``` <!DOCTYPE html> <html> <head> </head> <body> <script> (async() => { function screenShotSelection() { const div = document.createElement("div"); const styles = { background: "transparent", display: "block", position: "absolute", outline: "thin solid gold", width: "100px", height: "100px", left: "calc(50vw)", top: "calc(50vh)", overflow: "auto", resize: "both", cursor: "move", zIndex: 100 }; div.id = "screenShotSelector"; div.ondragstart = e => { console.log(e); }; div.ondragend = e => { const { clientX, clientY } = e; e.target.style.left = clientX + "px"; e.target.style.top = clientY + "px"; } Object.assign(div.style, styles); div.draggable = true; document.body.appendChild(div); } const video = document.createElement("video"); video.controls = true; video.style.objectFit = "cover"; video.style.lineHeight = 0; video.style.fontSize = 0; video.style.margin = 0; video.style.border = 0; video.style.padding = 0; video.loop = true; video.src = "https://upload.wikimedia.org/wikipedia/commons/d/d9/120-cell.ogv"; document.body.insertAdjacentElement("afterbegin", video); video.addEventListener("play", async e => { screenShotSelection(); const bounding = document.getElementById("screenShotSelector").getBoundingClientRect(); const stream = await navigator.mediaDevices.getDisplayMedia({ video: {cursor:"never"} // has no effect at Chromium }); const [videoTrack] = stream.getVideoTracks(); const imageCapture = new ImageCapture(videoTrack); const osc = new OffscreenCanvas(100, 100); // dynamic const osctx = osc.getContext("2d"); screenShotSelector.addEventListener("dblclick", async e => { console.log(window.getComputedStyle(e.target).left, window.getComputedStyle(e.target).top); osctx.drawImage(await imageCapture.grabFrame(), parseInt(window.getComputedStyle(e.target).left), parseInt(window.getComputedStyle(e.target).top), 100, 100, 0, 0, 100, 100); // dynamic console.log(bounding, URL.createObjectURL(await osc.convertToBlob())); videoTrack.stop(); video.pause(); }, { once: true }) }, { once: true }); })(); </script> </body> </html> ``` 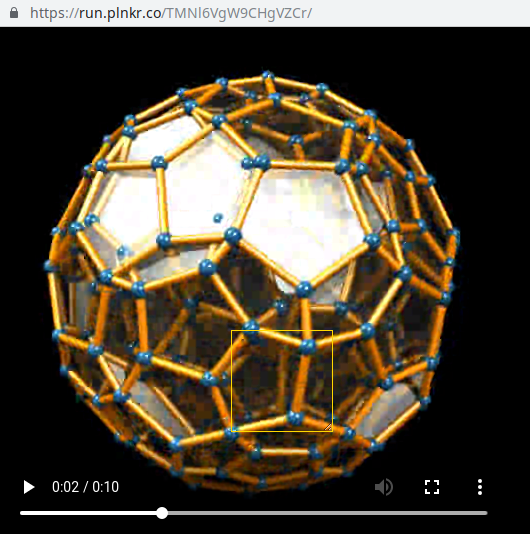 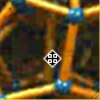 Ideally, specific for a screen shot (i.e., https://github.com/w3c/mediacapture-screen-share/issues/107) 1) once permission is granted to capture a screen, application of tab a draggable and resizable element having transparent background is appended to a fullscreen selection UI; 2) once a region has been selected for example using `dblclick` event on the draggable and resizable element a `MediaStreamTrack` is created and exists for the time to capture a single image is having the dimensions of the selection element, without the entire screen being captured then drawn again to resize to the element dimensions; the `MediaStreamTrack` is immediately stopped and ended once the single image is captured where the permission is not for a screen shot, but for a continuous media stream to be captured 1) only the selected region is captured (for example, the bounding client rectangle of the `<video>` element at the example code) not the entire screen; 2) all other `MediaStream` and `MediaStreamTrack` functionality remains the same -- GitHub Notification of comment by guest271314 Please view or discuss this issue at https://github.com/w3c/mediacapture-screen-share/issues/105#issuecomment-497998729 using your GitHub account
Received on Sunday, 2 June 2019 04:41:38 UTC