- From: André Gomes <notifications@github.com>
- Date: Thu, 29 Jul 2021 11:54:57 -0700
- To: WICG/webcomponents <webcomponents@noreply.github.com>
- Cc: Subscribed <subscribed@noreply.github.com>
- Message-ID: <WICG/webcomponents/issues/509/889381069@github.com>
I did some experiments with ElementObserver (https://stackblitz.com/edit/element-observer?devtoolsheight=44&file=index.js) in the spirit of [this component](https://webcomponents.dev/blog/all-the-ways-to-make-a-web-component/) and these are my findings: - it's confusing that `eo.observe(el)` triggers `connectedCallback` but `eo.disconnect(el)` doesn't trigger `disconnectedCallback`. - disconnectedCallback is triggered only if EO is connected and you remove the observed element from DOM. - I'd expect when I click the remove button the disconnect callback would be called and my div would revert to its original html content. - `eo.disconnect(el)` only means EO will no longer call your callbacks on DOM changes. - attr changed callback is called before connected callback when the element is added to dom with an observed attribute. - I think it makes sense to call both methods, but I'd expect connectedCallback first. - If connectedCallback comes first, I could put my initialization there (like the initial rendering of innerHTML), and use attr changed callback to re-render only the new values. - due to `MutationObserver`, if you make many changes to the elem at once (the mess button), your callbacks will be called multiple times and you'll have to be extra careful on how to react (difficult to determine what's the current state). - The main issue here is that the callbacks are called out of order and previous/current values become actually prev/final value. - If we can't guarantee the callback order and intermediate values, then we'd better swallow intermediate events and be notified only once with a summary of what happened (see https://github.com/mmacfadden/mutation-summary). Otherwise, you can imagine how confusing it is when you call **increment fn** but your callback says you went from 2 to 1 (•_•) ```js function messAround() { console.info('messing with the new one'); const el = document.getElementById('another-counter'); console.info('removing it from dom', el); el.remove(); console.info('changing its value', el); counter.inc(el); counter.dec(el); counter.dec(el); console.info('adding it back to dom', el); document.getElementById('app').append(el); } ``` 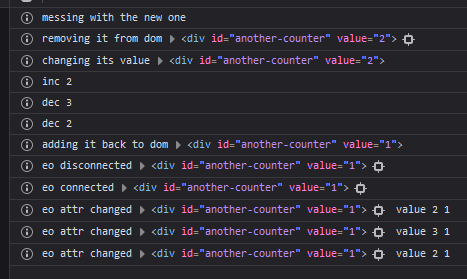 -- You are receiving this because you are subscribed to this thread. Reply to this email directly or view it on GitHub: https://github.com/WICG/webcomponents/issues/509#issuecomment-889381069
Received on Thursday, 29 July 2021 18:55:10 UTC