- From: Owen Buckley <notifications@github.com>
- Date: Wed, 01 Dec 2021 10:49:25 -0800
- To: whatwg/dom <dom@noreply.github.com>
- Cc: Subscribed <subscribed@noreply.github.com>
- Message-ID: <whatwg/dom/issues/831/983955120@github.com>
Hey there, hope it is OK to leave this here, but wanted to follow up from the great feedback provided during the recent TPAC sessions, wherein as part of the [Web Components Community Group](https://github.com/w3c/webcomponents-cg), we [presented on topics related to WCs](https://w3c.github.io/webcomponents-cg/), with Declarative Shadow DOM being a topic of interest to us. One of the feedback items shared with us was that it would be great to see an example of this in the wild, so I am back to share. 😃 #### Hardcoded In [this example](https://57xdk1noff.execute-api.us-east-1.amazonaws.com/prod/) I am just returning some hardcoded HTML to the browser. 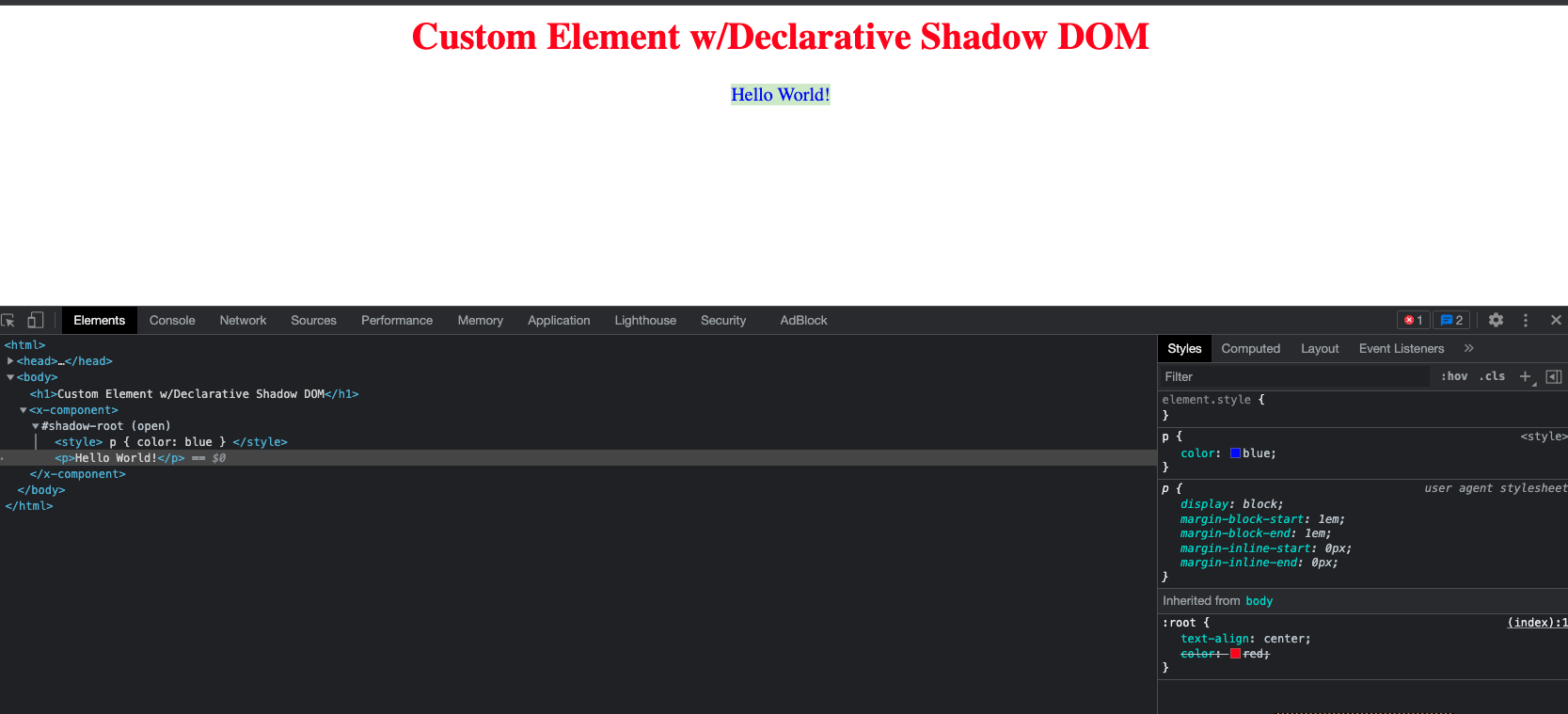 ```html <html> <head> <title>Declarative Shadow DOM Experiment</title> <style> :root { text-align: center; color: red; } </style> </head> <body> <h1>Custom Element w/Declarative Shadow DOM</h1> <x-component> <template shadowroot='open'> <style> p { color: blue } </style> <p>Hello World!</p> </template> </x-component> </body> </html> ``` #### Component Library (LitElement) [This example](https://v8ddb2guie.execute-api.us-east-1.amazonaws.com/prod/) uses [**Lit**](https://lit.dev/) and [its SSR capabilities](https://github.com/lit/lit/tree/main/packages/labs/ssr) to render WCs in NodeJS. I used the [Simple Greeting example](https://lit.dev/playground/) from their website. 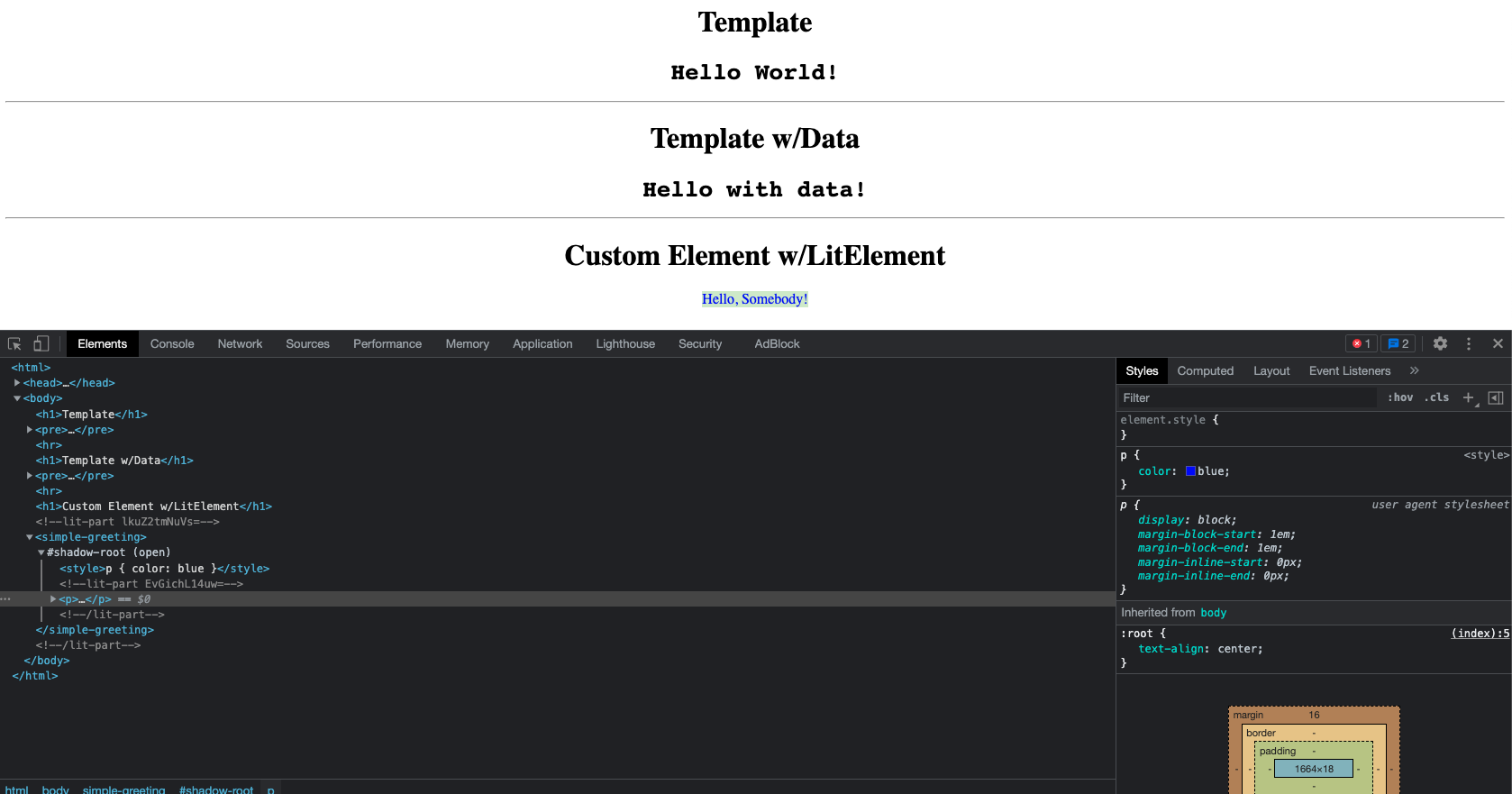 ```js // shim import first import { render } from '@lit-labs/ssr/lib/render-with-global-dom-shim.js'; import { Buffer } from 'buffer'; import { html } from 'lit'; import { Readable } from 'stream'; // these must be imported AFTER render-with-global-dom-shim.js import { helloTemplate } from '../public/scripts/hello-template.js'; import '../public/scripts/greeting-component.js'; async function streamToString (stream) { const chunks = []; for await (let chunk of stream) { chunks.push(Buffer.from(chunk)); } return Buffer.concat(chunks).toString("utf-8"); } const renderToHtml = async function() { const ssrResultHello = render(helloTemplate()); const ssrResultHelloData = render(helloTemplate('with data')); const ssrResultLitElement = render(html`<simple-greeting></simple-greeting>`); const resultStringHello = await streamToString(Readable.from(ssrResultHello)); const resultStringHelloData = await streamToString(Readable.from(ssrResultHelloData)); const resultStringLitElement = await streamToString(Readable.from(ssrResultLitElement)); return ` <html> <head> <title>Lit+Serverless Experiments</title> <style> :root { text-align: center; } </style> </head> <body> <h1>Template</h1> <pre>${resultStringHello}</pre> <hr> <h1>Template w/Data</h1> <pre>${resultStringHelloData}</pre> <hr> <h1>Custom Element w/LitElement</h1> ${resultStringLitElement} </body> </html> `; } export { renderToHtml } ``` ---- As a bit of a "bonus" to what I shared above, I thought it might worth mentioning that both of those examples are being rendered at "the edge" using serverless functions. I think it's pretty great to see modern specs, especially things as browser native as Shadow DOM and Custom Element, are now benefiting from the collective work of implementers, runtimes like **NodeJS**, and library authors like **Lit**, and it allows us to get more HTML out of our JS, and in most cases, not need to ship any JS at all. Users will be happy, that's for sure! 😅 > _You can observe that in both of those examples, there is no client side JS being shipped to the browser at all. Once your HTML gets properly cached in your CDN, it can be HTML all the way down._ 🐢 All in all, it's great to see all the hard work coming together and I hope the excitement and enthusiasm for web components and their potential usefulness in non browser runtimes can help guide this kind of work along. Declarative Shadow DOM is a a great stepping stone towards bringing the [full power of HTML](https://github.com/WICG/webcomponents/blob/gh-pages/proposals/Declarative-Custom-Elements-Strawman.md) to WCs and combined with proper hydration techniques, if there is the need to send some client side JS, it can be done so intelligently in a way that doesn't force unnecessary renders and page layout shifting or FOUC. Thanks, and let me know if you would like to see anything else in those demos and / or have any general feedback or thoughts. ✌️ -- You are receiving this because you are subscribed to this thread. Reply to this email directly or view it on GitHub: https://github.com/whatwg/dom/issues/831#issuecomment-983955120
Received on Wednesday, 1 December 2021 18:49:39 UTC